Using the Page Visibility API
The Page Visibility API allows developers to detect when a webpage becomes visible or hidden to users, enabling optimized content loading and efficient resource management. This API helps enhance user experience by adjusting application behavior based on visibility state changes, improving performance and engagement.
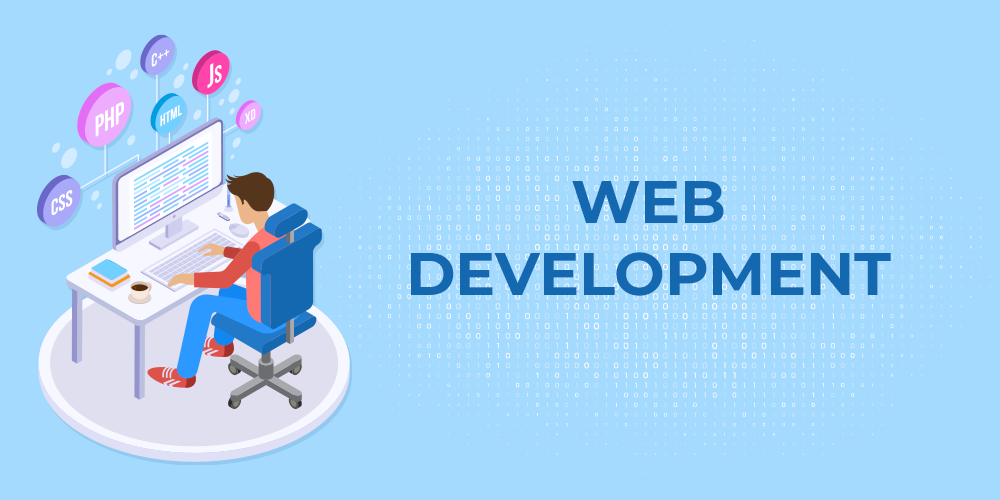
In the ever-evolving landscape of web development, ensuring optimal user experience is paramount. One of the tools that developers can leverage to enhance this experience is the Page Visibility API. This API allows web applications to detect when a page is visible to the user or hidden, which can be crucial for managing resources and improving performance. This guide will delve into the benefits of using the Page Visibility API, how it works, and its practical applications in modern web development.
Understanding the Page Visibility API
The Page Visibility API provides a way for developers to determine the visibility state of a web page. This means it can detect whether the user is actively viewing the page or if it is in the background. The API is part of the broader set of web standards that aim to make web applications more responsive and user-friendly.
The primary benefit of this API is its ability to help developers optimize the performance of their applications. By knowing when a page is not visible, developers can reduce the consumption of system resources, such as CPU and network bandwidth, which leads to more efficient applications and better user experiences.
How the Page Visibility API Works
The Page Visibility API works by providing an event that fires when the visibility state of a page changes. The API exposes the document.visibilityState
property and the visibilitychange
event, which developers can use to respond to changes in visibility.
Here’s a basic overview of how it operates:
-
Visibility States: The
document.visibilityState
property can have one of several values:visible
: The page is currently visible to the user.hidden
: The page is not visible to the user (e.g., it is in a background tab or minimized).prerender
: The page is being pre-rendered and is not visible yet.
-
Visibility Change Event: The
visibilitychange
event is triggered whenever the visibility state of the page changes. Developers can listen for this event to execute specific actions based on whether the page is visible or hidden.
Implementing the Page Visibility API
Implementing the Page Visibility API in your web application is straightforward. Here’s a simple example demonstrating how you can use the API to pause and resume a video based on the page visibility state:
if (document.visibilityState === 'hidden') {
// Pause the video if the page is hidden
document.querySelector('video').pause();
} else {
// Resume the video if the page becomes visible
document.querySelector('video').play();
}
});
document.addEventListener('visibilitychange', function() { if (document.visibilityState === 'hidden') { // Pause the video if the page is hidden document.querySelector('video').pause(); } else { // Resume the video if the page becomes visible document.querySelector('video').play(); } });
In this example, the visibilitychange
event listener checks the document.visibilityState
property to determine whether the page is visible or not. Depending on the state, it either pauses or plays the video.
Practical Applications of the Page Visibility API
The Page Visibility API can be applied in various scenarios to enhance user experience and optimize performance:
1. Optimizing Resource Usage
One of the key applications of the Page Visibility API is to optimize resource usage. For example, you can pause background tasks, stop animations, or halt network requests when the user is not actively viewing the page. This not only conserves system resources but also improves the overall efficiency of your web application.
2. Improving User Engagement
By leveraging the Page Visibility API, you can create more engaging experiences for users. For instance, you might choose to pause a game when the user navigates away from the page and resume it when they return. This ensures that users don't miss important moments and keeps them engaged with your content.
3. Enhancing Web Performance
The Page Visibility API allows you to manage performance more effectively by reducing unnecessary computations and operations when the page is not visible. For example, you can defer the loading of non-essential resources until the page becomes visible again, leading to faster load times and a smoother user experience.
4. Managing User Notifications
Web applications that provide notifications can use the Page Visibility API to manage how and when notifications are displayed. For example, you can choose to delay notifications or modify their behavior based on whether the user is actively viewing the page. This can help prevent users from being overwhelmed by notifications when they are not actively engaged.
Best Practices for Using the Page Visibility API
To make the most out of the Page Visibility API, consider the following best practices:
1. Avoid Overloading the Event Listener
While the Page Visibility API is powerful, it's essential to avoid overloading the visibilitychange
event listener with too many tasks. Make sure to keep the operations performed in response to visibility changes minimal and efficient to avoid potential performance issues.
2. Test Across Different Browsers
Different browsers may have varying levels of support for the Page Visibility API. Ensure you test your implementation across different browsers and devices to ensure consistent behavior and performance.
3. Use Fallbacks When Necessary
In some cases, the Page Visibility API may not be supported or may behave differently across browsers. Implement fallbacks or alternative solutions to handle scenarios where the API is not available or functioning as expected.
FAQ
Q1: What browsers support the Page Visibility API?
The Page Visibility API is supported by most modern browsers, including Chrome, Firefox, Safari, Edge, and Opera. However, it is always a good practice to check the current compatibility on resources like MDN Web Docs or Can I Use.
Q2: Can I use the Page Visibility API on mobile devices?
Yes, the Page Visibility API is supported on mobile devices as well. It can be used to enhance the performance and user experience of mobile web applications by managing resource usage based on page visibility.
Q3: What should I do if the Page Visibility API is not supported in a particular browser?
If the Page Visibility API is not supported, consider implementing alternative solutions or fallbacks. You can use standard techniques to manage resources and optimize performance, such as manual checks or timers, to ensure a good user experience.
Q4: How can I check if the Page Visibility API is supported in the user's browser?
You can check for support by verifying if the document.hidden
property exists. For example:
// Page Visibility API is supported
} else {
// Page Visibility API is not supported
}
if (typeof document.hidden !== "undefined") { // Page Visibility API is supported } else { // Page Visibility API is not supported }
Q5: Are there any performance implications of using the Page Visibility API?
The Page Visibility API itself has minimal performance implications. However, how you use it can impact performance. Ensure that the operations you perform in response to visibility changes are efficient and do not overload the event listener.
The Page Visibility API is a valuable tool for developers aiming to create more responsive and resource-efficient web applications. By understanding how it works and implementing it effectively, you can enhance user experience and optimize performance in your web projects.
Get in Touch
Website – https://www.webinfomatrix.com
Mobile - +91 9212306116
Whatsapp – https://call.whatsapp.com/voice/9rqVJyqSNMhpdFkKPZGYKj
Skype – shalabh.mishra
Telegram – shalabhmishra
Email - info@webinfomatrix.com
What's Your Reaction?

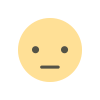
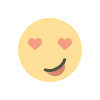
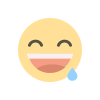
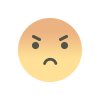
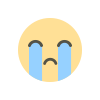
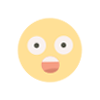